How Developers Can Enhance Their Debugging Competencies By Gustavo Woltmann
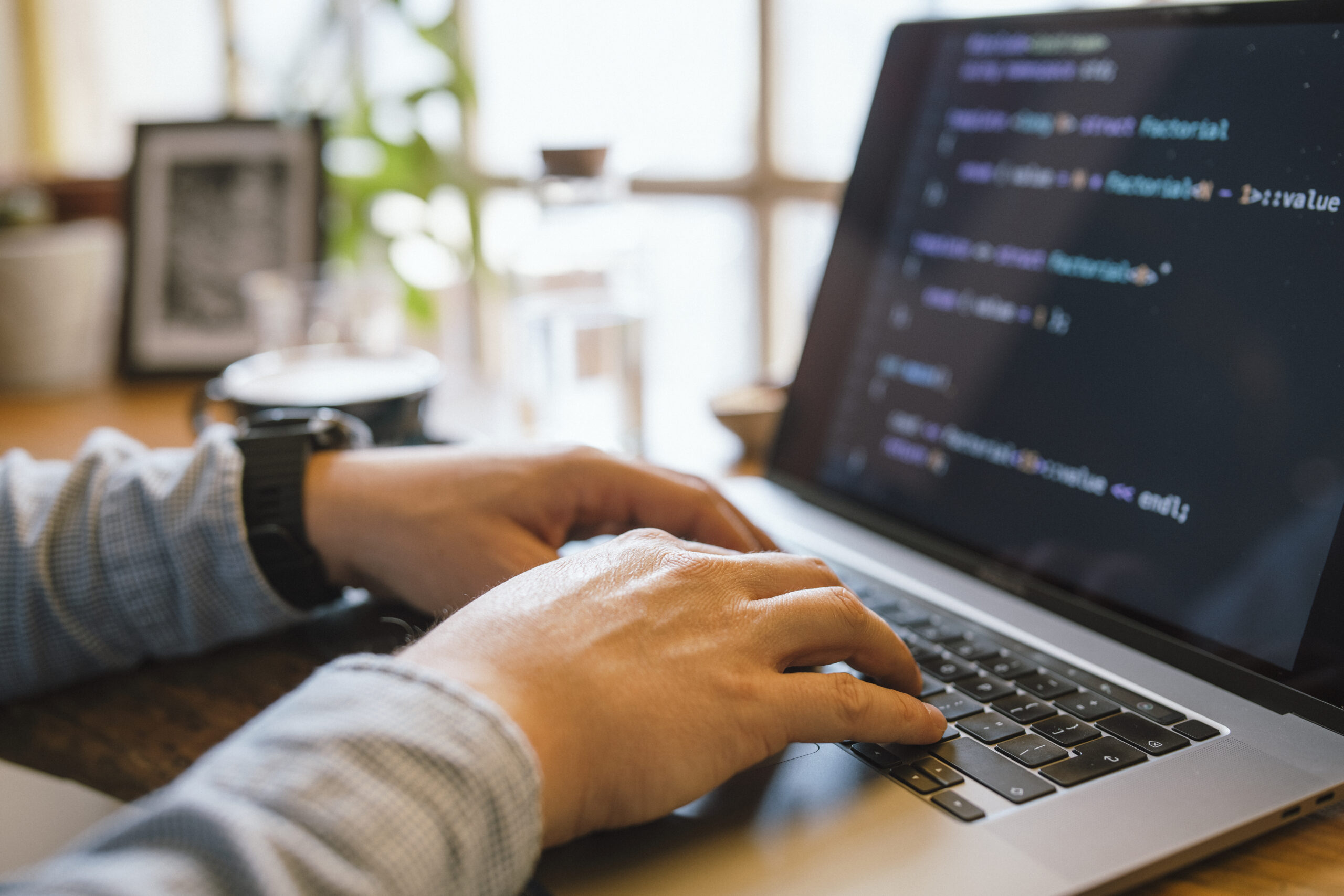
Debugging is Probably the most vital — yet usually neglected — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to resolve troubles successfully. Irrespective of whether you're a newbie or possibly a seasoned developer, sharpening your debugging capabilities can help save several hours of irritation and dramatically improve your efficiency. Here are a number of methods to aid builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Equipment
One of several quickest techniques developers can elevate their debugging skills is by mastering the tools they use each day. While crafting code is a person Component of growth, realizing how you can connect with it proficiently for the duration of execution is equally vital. Modern-day advancement environments come Geared up with effective debugging abilities — but quite a few developers only scratch the area of what these equipment can do.
Just take, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion builders. They let you inspect the DOM, observe network requests, look at real-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can switch frustrating UI concerns into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate units like Git to know code heritage, obtain the precise instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your equipment suggests likely further than default settings and shortcuts — it’s about building an personal familiarity with your progress natural environment to make sure that when challenges crop up, you’re not shed in the dark. The greater you know your tools, the greater time you could expend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most vital — and often ignored — steps in helpful debugging is reproducing the condition. In advance of jumping in to the code or making guesses, builders will need to produce a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about wasted time and fragile code adjustments.
The first step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you might have, the simpler it results in being to isolate the exact disorders beneath which the bug occurs.
When you finally’ve collected ample info, endeavor to recreate the issue in your neighborhood atmosphere. This might imply inputting a similar info, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automated assessments that replicate the sting circumstances or condition transitions associated. These exams not simply help expose the trouble but also avoid regressions Down the road.
Occasionally, The problem may very well be surroundings-precise — it'd take place only on selected functioning systems, browsers, or below distinct configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t simply a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Having a reproducible scenario, You can utilize your debugging equipment a lot more properly, take a look at probable fixes safely and securely, and converse far more Evidently with all your workforce or buyers. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Read through and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then observing them as annoying interruptions, developers should master to take care of error messages as direct communications within the process. They typically tell you exactly what transpired, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the very first line and immediately start out creating assumptions. But deeper during the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and stage you towards the responsible code.
It’s also valuable to understand the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically hasten your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Verify relevant log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent equipment within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood while not having to pause execution or phase in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data in the course of advancement, Information for general situations (like prosperous start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant data. Too much logging can obscure vital messages and decelerate your program. Concentrate on key situations, condition modifications, enter/output values, and significant choice details as part of your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t attainable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging strategy, you could reduce the time it requires to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Like a Detective
Debugging is not simply a technological job—it's a sort of investigation. To correctly recognize and deal with bugs, builders should technique the procedure similar to a detective solving a mystery. This attitude allows break down sophisticated troubles into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Just like a detective surveys a crime scene, obtain just as much applicable information as you are able to with out jumping to conclusions. Use logs, check situations, and consumer studies to piece jointly a transparent photo of what’s occurring.
Following, kind hypotheses. Request by yourself: What can be producing this behavior? Have any changes recently been produced towards the codebase? Has this issue occurred before less than identical situation? The purpose is always to narrow down possibilities and detect probable culprits.
Then, examination your theories systematically. Make an effort to recreate the trouble in a managed natural environment. For those who suspect a certain operate or component, isolate it and confirm if The problem persists. Similar to a detective conducting interviews, check with your code inquiries and Allow the results direct you closer to the reality.
Pay out close notice to modest particulars. Bugs normally cover in the minimum expected sites—just like a lacking semicolon, an off-by-just one error, or a race issue. Be thorough and patient, resisting the urge to patch The problem with out thoroughly comprehension it. Temporary fixes may well conceal the actual issue, just for it to resurface later.
And finally, maintain notes on That which you tried and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks understand your reasoning.
By pondering similar to a detective, developers can sharpen their analytical expertise, tactic issues methodically, and grow to be more practical at uncovering concealed issues in sophisticated units.
Create Assessments
Writing exams is among the simplest approaches to increase your debugging competencies and overall improvement effectiveness. Assessments not simply help catch bugs early but additionally serve as a safety Internet that provides you self esteem when building variations to your codebase. A nicely-tested application is easier to debug because it permits you to pinpoint specifically the place and when a challenge takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can promptly expose no matter if a certain bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know exactly where to appear, considerably decreasing the time spent debugging. Device assessments are Specially beneficial for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclusion-to-conclude exams into your workflow. These assist ensure that several areas of your application work jointly easily. They’re especially practical for catching bugs that arise in sophisticated systems with many elements or services interacting. If a little something breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to think critically about your code. To check a characteristic properly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to better code framework and much less bugs.
When debugging a problem, crafting a failing examination that reproduces the bug can be a strong starting point. After the take a look at fails regularly, you may concentrate on repairing the bug and check out your check move when The difficulty is resolved. This technique makes certain that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the irritating guessing match right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your mind, decrease aggravation, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for also extended, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hrs earlier. Within this state, your Mind will become a lot less successful at challenge-fixing. A short walk, a espresso split, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also aid prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer mentality. You could possibly all of a sudden notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nonetheless it actually contributes to faster and simpler debugging in the long run.
Briefly, taking breaks will not be a sign of weak point—it’s a sensible method. It offers your brain Area to breathe, increases your perspective, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each and every bug you come upon is more than just A brief setback—It can be a possibility to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each one can teach you one read more thing precious for those who make an effort to mirror and review what went Improper.
Start off by inquiring you a couple of crucial inquiries when the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or comprehension and make it easier to Make more robust coding behaviors transferring ahead.
Documenting bugs can be a fantastic routine. Hold a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you learned. As time passes, you’ll start to see styles—recurring difficulties or prevalent problems—which you could proactively keep away from.
In group environments, sharing Whatever you've discovered from the bug with all your friends could be Specifically potent. Whether or not it’s via a Slack concept, a short generate-up, or A fast information-sharing session, aiding Other people steer clear of the very same concern boosts team effectiveness and cultivates a stronger Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your growth journey. After all, many of the very best builders aren't those who create great code, but those that repeatedly discover from their faults.
In the end, Just about every bug you repair provides a new layer in your talent set. So following time you squash a bug, have a moment to mirror—you’ll appear away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — nevertheless the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to be better at Everything you do.